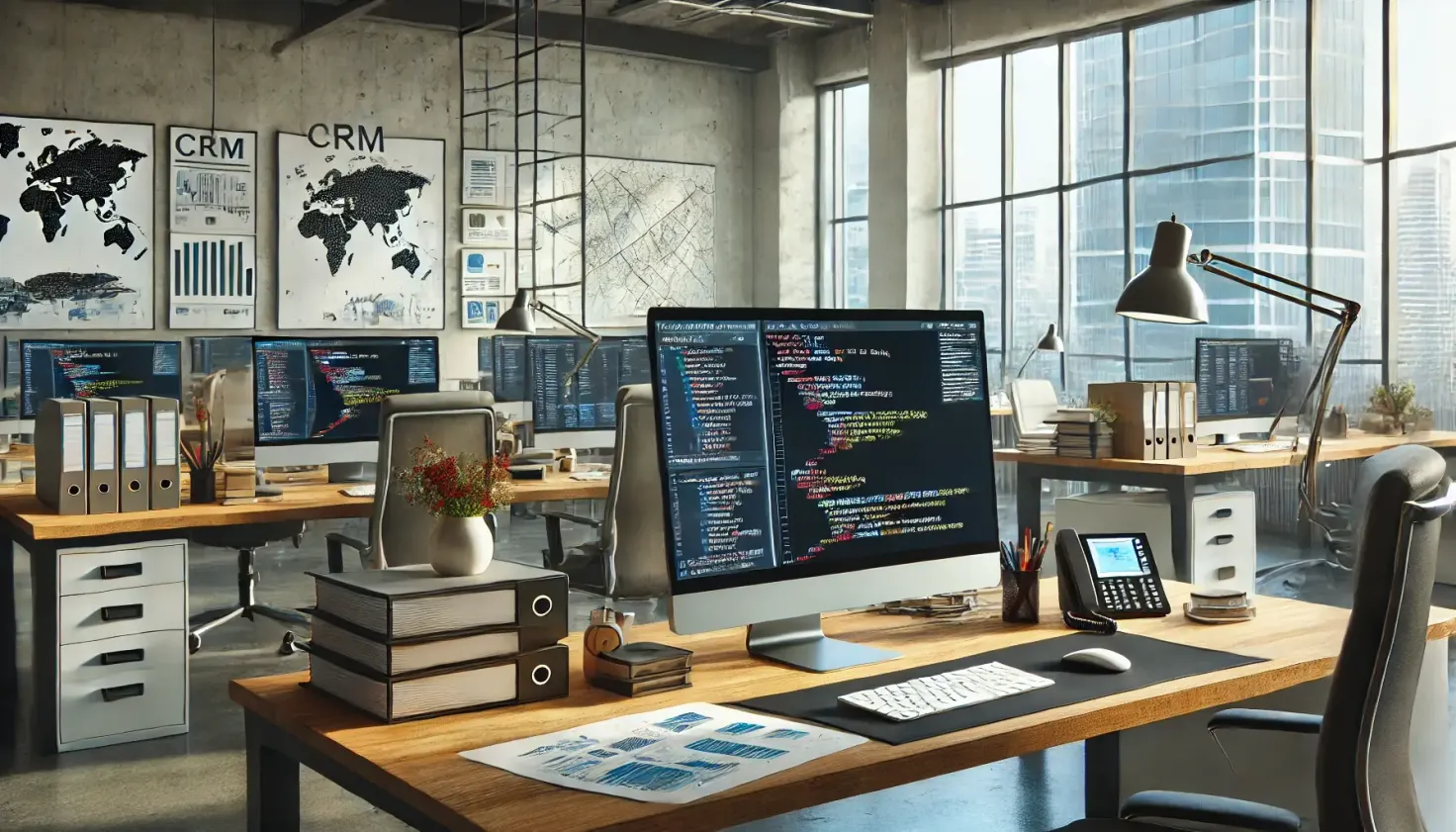
Extending SugarCRM: Using ActionFileMap for Custom Actions
In SugarCRM, developers frequently need to add custom actions that extend the application's default functionality. One way to do this is by using the ActionFileMap
extension, which allows you to map actions directly to PHP files for execution. This blog will explore how ActionFileMap
works and when it is most useful in creating upgrade-safe customizations.
What is ActionFileMap?
The ActionFileMap
extension is a feature of SugarCRM that allows developers to map specific actions directly to PHP files. This means that, rather than mapping an action to a predefined view (like detailview
or editview
), you can route an action to a custom PHP file. This can be useful for actions that perform backend logic, trigger automated processes, or integrate with external systems where a full view rendering may not be necessary.
Why Use ActionFileMap?
Using ActionFileMap
has several benefits:
- Direct File Mapping: Instead of creating a view file that is routed through a controller,
ActionFileMap
allows you to specify a standalone PHP file, reducing overhead. - Custom Logic Without Views: For actions that don't require user interaction (like background processes), mapping to a file can be more efficient.
- Upgrade-Safe Customization: Adding custom files in the
custom
directory, combined with theActionFileMap
, keeps your code upgrade-safe and separate from SugarCRM's core files.
Setting Up ActionFileMap
To set up an ActionFileMap
entry, you'll create a file within the custom/Extension/modules/<module>/Ext/ActionFileMap/
directory. This file will define mappings between action names and custom PHP files.
- Define the Action in ActionFileMap
<?php
$action_file_map['custom_action'] = 'custom/modules/<module>/customAction.php';
- Implement the Custom Action Logic
<?php
if (!defined('sugarEntry') || !sugarEntry) die('Not A Valid Entry Point');
class CustomActionHandler
{
public function execute()
{
// Custom logic goes here
echo "This is the result of the custom action!";
}
}
$handler = new CustomActionHandler();
$handler->execute();
- Perform Quick Repair and Rebuild
After creating and mapping the action file, perform a Quick Repair and Rebuild from the SugarCRM Admin panel. This ensures the system recognizes the new mapping and includes it in the application's action routing.
Benefits of Using ActionFileMap
- Direct Control Over Action Files: With
ActionFileMap
, you can directly map actions to backend logic files, bypassing the need for view rendering when it's unnecessary. - Efficiency for Background Processes: For processes that don't require user interaction, using a PHP file directly is often more efficient and lightweight.
- Clear Separation from Core Code: Storing action mappings and custom logic files in
custom/
keeps modifications upgrade-safe and organized.
Example Use Case: Exporting Custom Data
- Map the Export Action
<?php
$action_file_map['export_custom_data'] = 'custom/modules/<module>/exportData.php';
- Define Export Logic in the PHP File
<?php
if (!defined('sugarEntry') || !sugarEntry) die('Not A Valid Entry Point');
class ExportData
{
public function execute()
{
// Custom export logic, e.g., generating CSV data
header('Content-Type: text/csv');
header('Content-Disposition: attachment; filename="export.csv"');
echo "Column1,Column2,Column3\n";
echo "Data1,Data2,Data3\n";
}
}
$export = new ExportData();
$export->execute();
Summary
ActionFileMap
in SugarCRM is an efficient way to map custom actions directly to PHP files, making it ideal for backend logic or actions that don't require a user interface. Using ActionFileMap
is upgrade-safe and keeps customizations organized, modular, and easy to maintain. Whether you're creating an API integration, automating workflows, or handling data exports, ActionFileMap
provides a powerful tool for SugarCRM developers looking to extend the application's functionality.