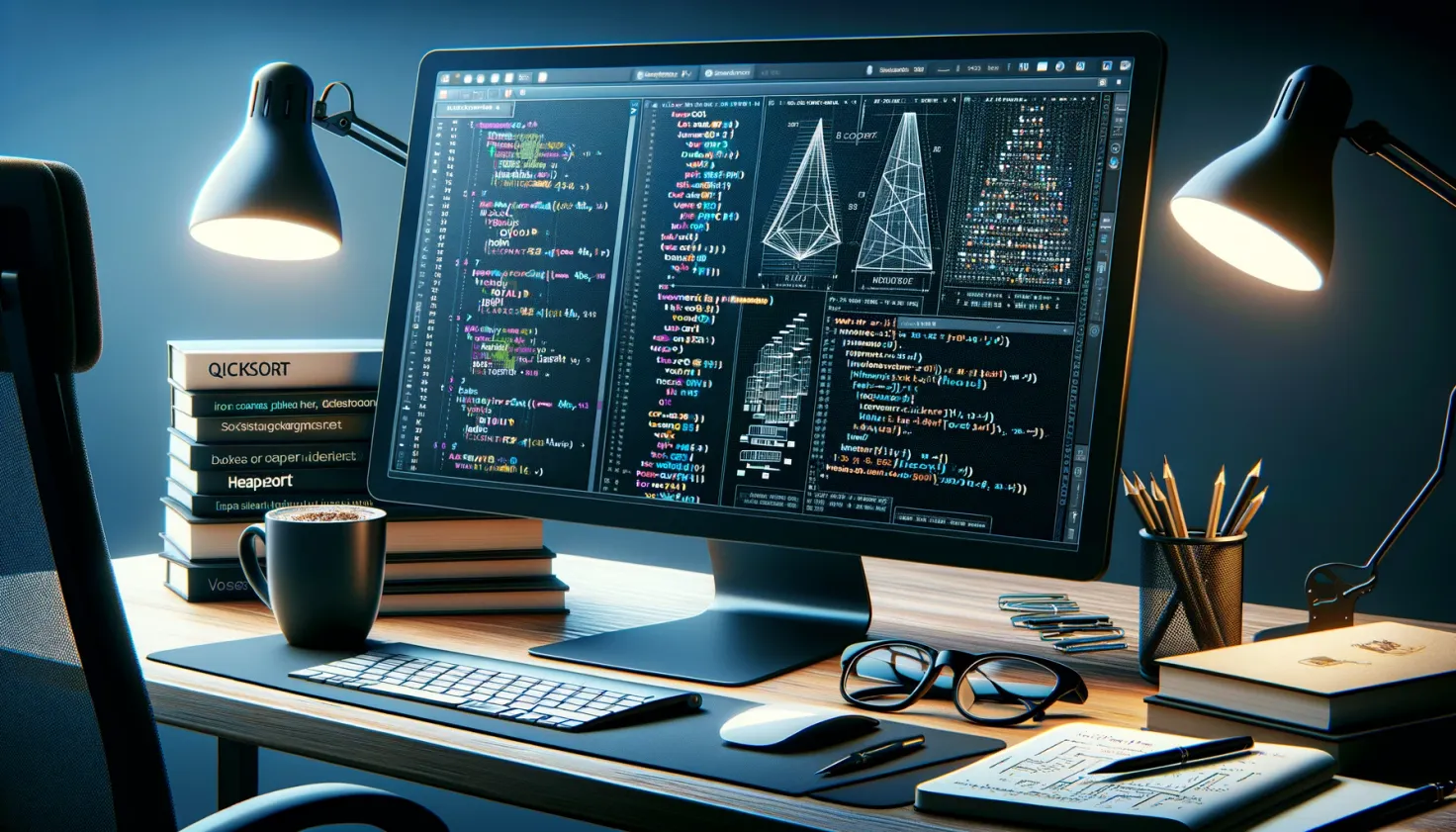
The Top Three Sorting Algorithms You Need to Know for Coding Interviews
When preparing for coding interviews, understanding sorting algorithms is crucial. Not only do they form the foundation of algorithmic thinking, but they also frequently appear in interview questions either directly or as part of more complex problem-solving tasks. In this article, we'll delve into three essential sorting algorithms: QuickSort, MergeSort, and HeapSort. We'll explore their mechanisms, analyze their complexities, and discuss their practical applications and how to approach related interview questions.
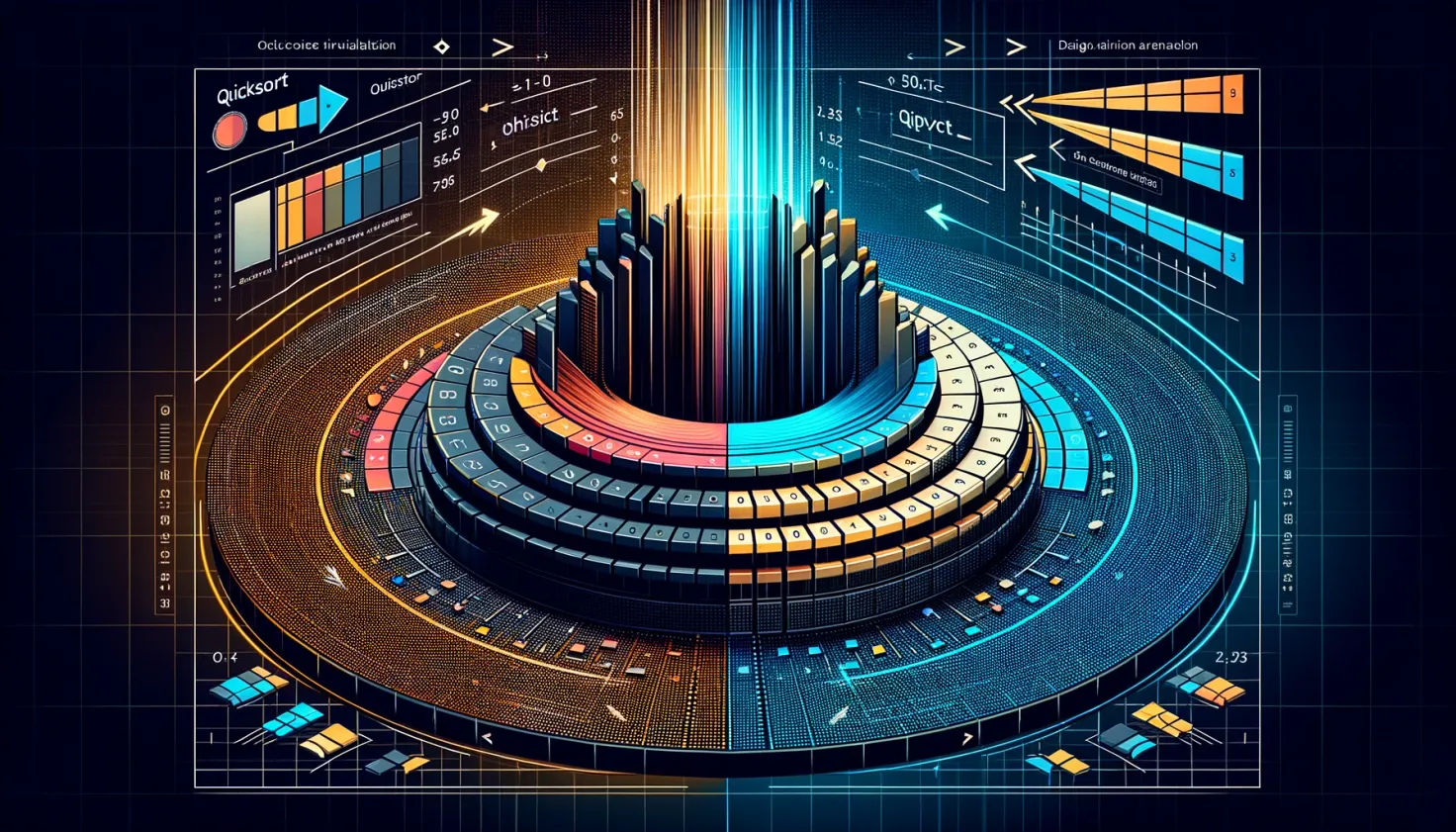
QuickSort: The Efficient Divider
Overview: QuickSort is a fast and efficient sorting algorithm that operates on the divide-and-conquer strategy. It selects an element as a pivot and partitions the array around the pivot, ensuring elements on one side are less than the pivot and those on the other are greater.
Time Complexity: On average, QuickSort operates in O(n log n) time, making it incredibly efficient for most datasets. However, its worst-case performance is O(n^2), which can occur when the smallest or largest element is always chosen as the pivot.
Space Complexity: QuickSort has a space complexity of O(log n) due to the recursive stack calls.
Pros and Cons: Its main advantage is its superior average-case performance and low memory usage (in-place sorting). However, its worst-case scenario and the choice of pivot can be problematic if not managed well.
Practical Applications: QuickSort is widely used in libraries and applications for its general efficiency and simplicity, especially where average-case performance is prioritized.
Interview Tips: Be prepared to discuss the choice of pivot and how it affects the algorithm's performance. Implementing QuickSort in an interview might require you to write a partitioning function, a critical component to get right.
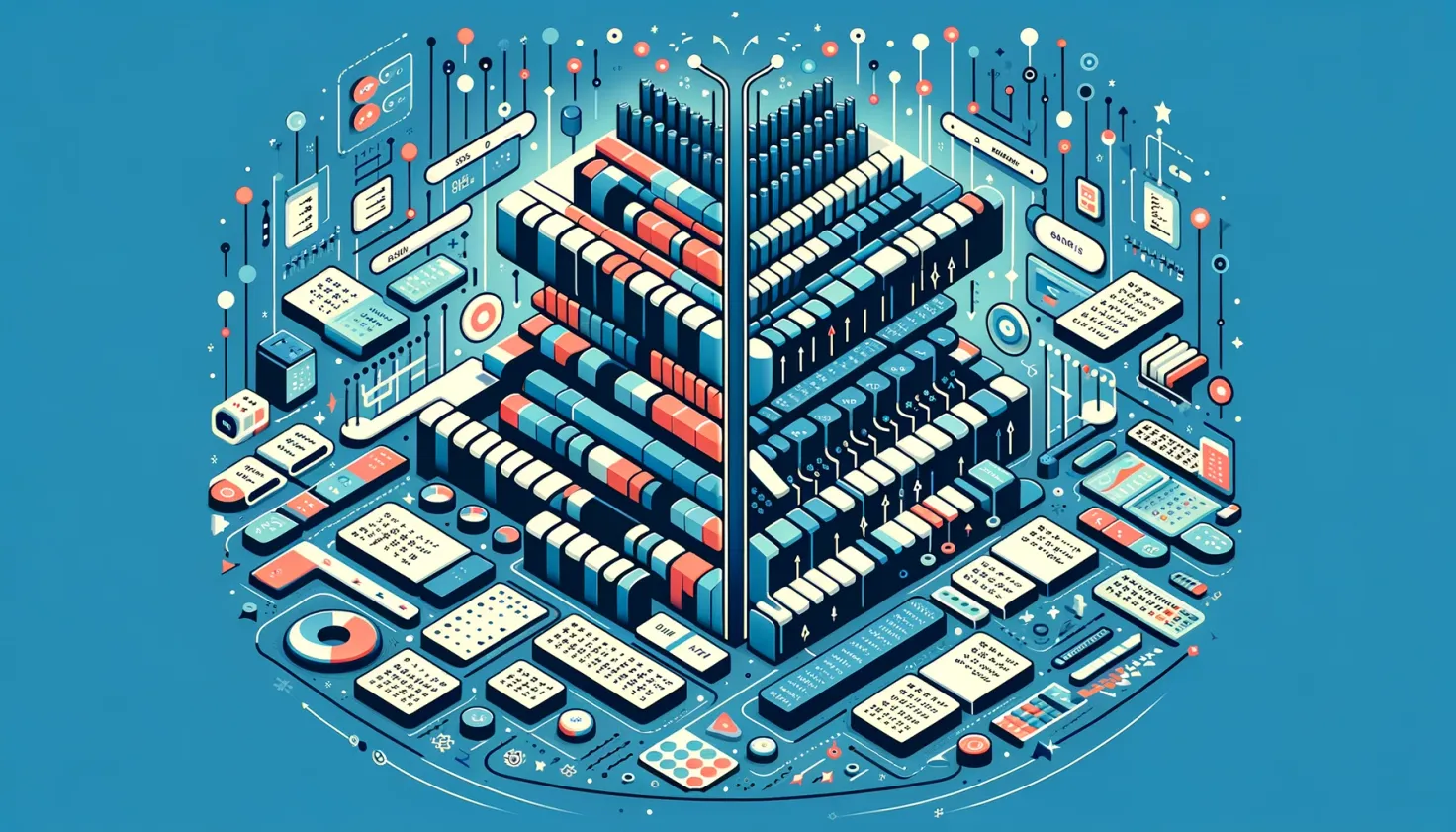
MergeSort: The Reliable Performer
Overview: MergeSort is a stable sorting algorithm that divides the array into halves, sorts each half, and then merges them back together. It guarantees a running time of O(n log n) in all cases, making it highly reliable.
Time Complexity: Its time complexity is always O(n log n), whether in the best, average, or worst-case scenarios.
Space Complexity: MergeSort requires additional space for storing the temporary arrays used during the merge process, leading to a space complexity of O(n).
Pros and Cons: The algorithm's main advantage is its stable sorting and consistent performance across all types of data sets. However, its higher space requirement can be a drawback in memory-constrained environments.
Practical Applications: MergeSort is preferred in scenarios requiring stable sorting, such as sorting linked lists or when working with large datasets where the data cannot be loaded entirely into memory.
Interview Tips: Understanding the merge process is key. Be ready to implement or discuss the merging of two sorted arrays, a common interview question that tests basic understanding of the algorithm.
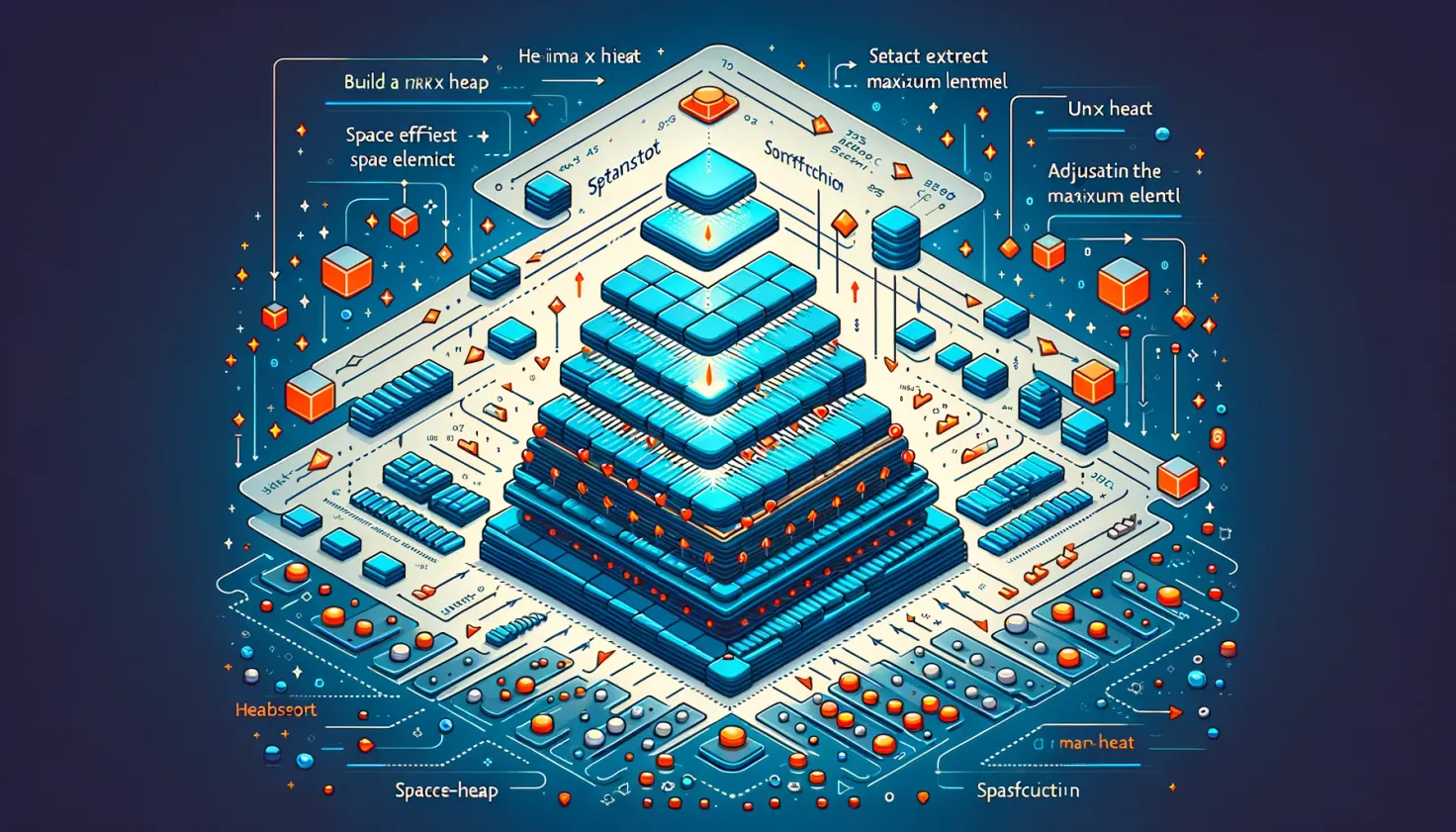
HeapSort: The Space-Efficient Sorter
Overview: HeapSort sorts an array by building a heap from the input data, then repeatedly extracting the maximum element from the heap and adjusting the heap accordingly.
Time Complexity: HeapSort operates in O(n log n) time for all cases, making it efficient and predictable.
Space Complexity: One of HeapSort's significant advantages is its in-place sorting capability, which leads to a space complexity of O(1), excluding the stack space used for recursion in heap construction.
Pros and Cons: The primary benefit of HeapSort is its memory efficiency. However, it is not a stable sort, and its real-world performance can lag behind QuickSort and MergeSort due to cache inefficiency.
Practical Applications: HeapSort is useful when memory space is limited, and stability is not a concern. It's also foundational for understanding priority queues and heap-based data structures.
Interview Tips: Be prepared to discuss how a binary heap works and how HeapSort can be implemented using this data structure. Demonstrating the heapify process and the concept of in-place sorting can be crucial.
Conclusion
QuickSort, MergeSort, and HeapSort each offer unique advantages and are pivotal for any software developer's interview preparation. Understanding these algorithms' inner workings, their complexities, and their trade-offs prepares you not just to answer interview questions but also to make informed decisions about which algorithm to use in different scenarios.
When discussing these algorithms in interviews, clarity of thought, understanding of their mechanics, and the ability to analyze their complexities will set you apart. Remember, the goal is not just to memorize these algorithms but to understand their principles, as this knowledge will enable you to tackle a wide array of problems more effectively.
Happy coding, and good luck with your interviews!